The Power of Focusing on Goals: Embracing a Declarative Mindset
Introduction
In software development and project management, the way we tackle problems can make a huge difference. Two main approaches—declarative and imperative—offer distinct paths. While both have their strengths, taking a declarative approach often brings more flexibility and innovation.
In this article, we'll dive into the perks of having a declarative mindset, especially for product owners who set acceptance criteria. We'll explore how this mindset encourages creativity and efficiency, using React
as a tech example. By defining the goals and letting the experts figure out the "how," you open the door to endless possibilities and optimizations.
Key Takeaways
- Declarative Mindset: Focus on the goals rather than the "how" to unlock creativity and innovation.
- React: A great example of how declarative UI can boost performance and flexibility.
- Optimization: Declarative methods make it easier to optimize and adapt over time.
- Expert Utilization: Let your team shine by specifying the outcomes, not the methods.
Declarative vs. Imperative: What’s the Difference?
Declarative Mindset
A declarative mindset is all about stating what you want without dictating how to achieve it. This lets your team use their skills to find the best solutions. For instance, a product owner might specify that a user should be able to log in securely (the goal) but leave the implementation details (the "how") to the development team.
Imperative Mindset
In contrast, an imperative mindset involves giving specific instructions on how to achieve a goal. This can limit creativity and restrict the team's ability to optimize and improve the solution over time. An imperative approach might involve telling the developers exactly which authentication methods to use, potentially missing out on more secure or efficient alternatives.
React: A Declarative Approach to UI Development
The Benefits of Declarative UI
React
exemplifies the power of a declarative approach in UI development. By describing the desired state of the UI and letting React handle the rendering and updates, developers can focus on what the UI should look like and how it should behave, rather than getting bogged down in the specifics of DOM manipulation.
Example: Creating an Interactive Component
Let's create a simple button example with some interactivity using the useState
hook. We'll create a counter component that increments the count each time the button is clicked.
import React, { FC, useState } from 'react';
type CounterProps = {
initialCount: number;
};
const Counter: FC<CounterProps> = ({ initialCount }) => {
const [count, setCount] = useState(initialCount);
const handleIncrement = () => {
setCount(count + 1);
};
return (
<div>
<p>Current Count: {count}</p>
<button onClick={handleIncrement}>Increment</button>
</div>
);
};
export default Counter;
In this example:
- We declare a Counter component that takes an initialCount as a prop.
- We use the
useState
hook to manage the state of the count. - The
handleIncrement
function updates the state by incrementing the count. - The component renders the current count and a button that increments the count when clicked.
This simple example showcases the declarative nature of React
. We define the desired state and behavior, and React
takes care of updating the UI based on state changes.
Optimizing Performance and Flexibility
Declarative UI in React
allows for performance optimizations such as memoization and lazy loading. Additionally, it enables seamless transitions to technologies like React Native, extending the reach of your application to mobile platforms with minimal code changes.
Conclusion
Embracing a declarative, goal-oriented mindset can transform how you approach product development and software engineering. By focusing on the goals and leaving the "how" to your team of experts, you open the door to innovative solutions and continuous optimization. Technologies like React
showcase the power of declarative thinking, providing flexible, performant, and maintainable code. Shift your perspective, harness the power of focusing on the goals, and watch your projects thrive.
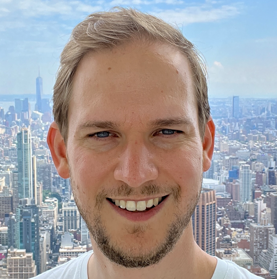
René Viering
Freelance Software Developer
Do you want to test your knowledge about the article?